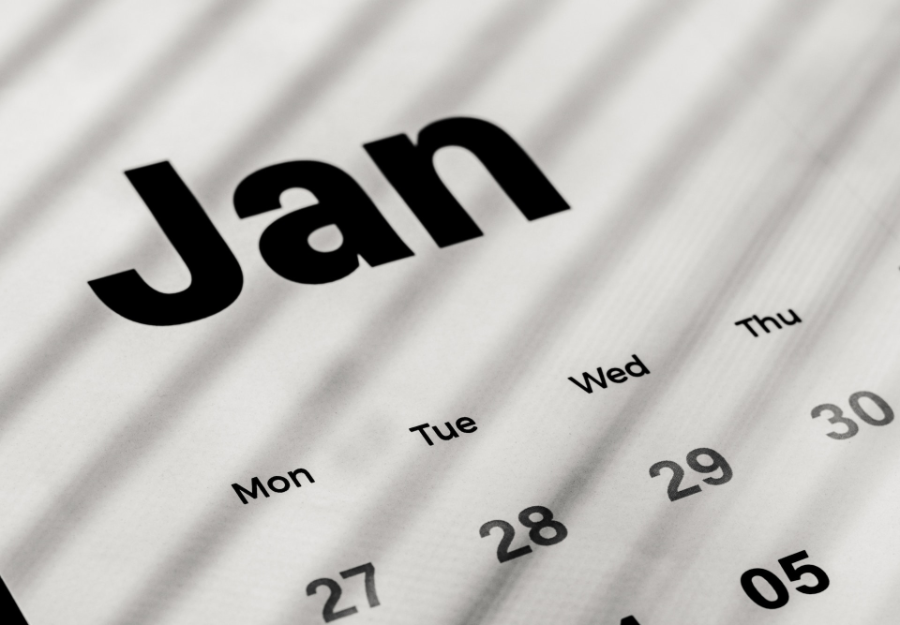
Do you have a moment?
Working with dates in native JavaScript can quickly become tedious and cumbersome. You have the standard Date object with a few different methods for constructing that object. Then you have a series of getXXX
and setXXX
methods that let you work with and manipulate various pieces of that Date object.
But what if you need to do more than that? What if you need to calculate future dates from this object? How do you display your date as a relative time like ‘4 hours ago’ or ‘3 weeks ago’. How do you compare two different Date objects? You could start down a long road toward building your own library of utility methods to answer some of these questions or … Enter moment.js.
Moment.js is a very small script (5k minified) that let’s you work with dates in a very logical way using a syntax that’s easy to read. This takes all of the guesswork out of Date manipulation.
Suppose you have a scenario in NetSuite where you need to calculate the revenue recognition start and end dates of an invoice. We have a business rule that says revenue recognition starts on the first day of the second month after the product ships and ends after 24 months. To do this with plain JavaScript, we’re looking at adding 60 days to the existing date, and then using setDate to put us back to the 1st of the month. For the end date, we can add 24 * 30 days from the start date to approximate the end date. But what about months with 31 days or 28 days? What about leap years? If you’re looking at this code later, is the intent immediately clear?
With moment.js, you can use a declarative set of methods to describe your business rule clearly.
var record = nlapiLoadRecord(‘invoice’, 12345);
var transactionDate = record.getFieldValue(‘trandate’);
var revRecStartDate = moment(transactionDate)
.add(‘months’, 2)
.startOf(‘month’)
.format(‘MM/DD/YYYY’);
var revRecEndDate = moment(revRecStartDate)
.add(‘months’, 24)
.startOf(‘month’)
.subtract(‘days’, 1)
.format(‘MM/DD/YYYY’);
In this example, we retrieve the transaction date from the current invoice. Then, using moment.js, I start with the transaction date, add 2 months, go to the first of the month.
For the end date, I begin with the start date, add 24 months, go to the first of the month, then subtract 1 day (without the last step, revenue recognition would include an extra month).
This is much more clear about what we’re trying to do. It’s easy to read when you come back to this code months later. We don’t have to worry about long and short months, leap years or estimations of any kind.
Some of the other things you can do with moment.js:
fromNow
orfromX
to display dates in a ‘3 hours ago’ format.local
or .utc
to switch back and forth between local and UTC dates.calendar
to display dates as ‘Last Monday’ or ‘Tomorrow’.diff
to get the difference between twomoment
objects.isBefore
,isAfter
,isSame
to comparemoment
objects.
The list goes on. You can read all of the great documentation here. You can download the file directly or add it as a NuGet package with:
Install-Package Moment.js